Description
You are given an m x n
matrix board
containing letters 'X'
and 'O'
, capture regions that are surrounded:
- Connect: A cell is connected to adjacent cells horizontally or vertically.
- Region: To form a region connect every
'O'
cell. - Surround: The region is surrounded with
'X'
cells if you can connect the region with'X'
cells and none of the region cells are on the edge of theboard
.
To capture a surrounded region, replace all 'O'
s with 'X'
s in-place within the original board. You do not need to return anything.
Example 1:
Input: board = [["X","X","X","X"],["X","O","O","X"],["X","X","O","X"],["X","O","X","X"]]
Output: [["X","X","X","X"],["X","X","X","X"],["X","X","X","X"],["X","O","X","X"]]
Explanation:
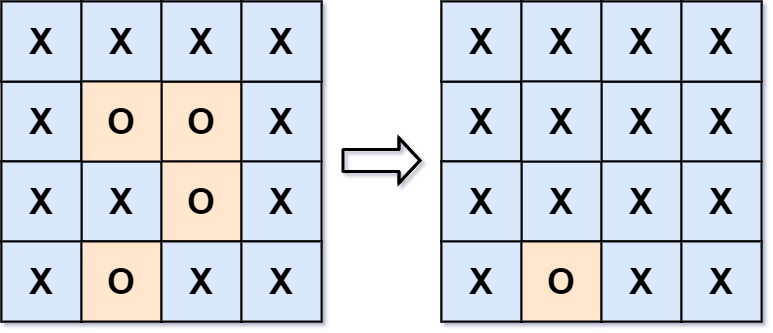
In the above diagram, the bottom region is not captured because it is on the edge of the board and cannot be surrounded.
Example 2:
Input: board = [["X"]]
Output: [["X"]]
Constraints:
m == board.length
n == board[i].length
1 <= m, n <= 200
board[i][j]
is'X'
or'O'
.
Solution
Python3
class Solution:
def solve(self, board: List[List[str]]) -> None:
"""
Do not return anything, modify board in-place instead.
"""
rows, cols = len(board), len(board[0])
queue = collections.deque()
for x in range(rows):
for y in [0, cols - 1]:
if board[x][y] == 'O':
board[x][y] = 'G'
queue.append((x, y))
for x in [0, rows - 1]:
for y in range(cols):
if board[x][y] == 'O':
board[x][y] = 'G'
queue.append((x, y))
while queue:
x, y = queue.popleft()
for dx, dy in [(x + 1, y), (x - 1, y), (x, y + 1), (x, y - 1)]:
if 0 <= dx < rows and 0 <= dy < cols and board[dx][dy] == 'O':
board[dx][dy] = 'G'
queue.append((dx, dy))
for x in range(rows):
for y in range(cols):
if board[x][y] == 'O':
board[x][y] = 'X'
elif board[x][y] == 'G':
board[x][y] = 'O'